3 gen_statem Behavior
This section is to be read with the gen_statem(3) manual page in STDLIB, where all interface functions and callback functions are described in detail.
This is a new behavior in Erlang/OTP 19.0. It has been thoroughly reviewed, is stable enough to be used by at least two heavy OTP applications, and is here to stay. Depending on user feedback, we do not expect but can find it necessary to make minor not backward compatible changes into Erlang/OTP 20.0.
3.1 Event-Driven State Machines
Established Automata Theory does not deal much with how a state transition is triggered, but assumes that the output is a function of the input (and the state) and that they are some kind of values.
For an Event-Driven State Machine, the input is an event that triggers a state transition and the output is actions executed during the state transition. It can analogously to the mathematical model of a Finite-State Machine be described as a set of relations of the following form:
State(S) x Event(E) -> Actions(A), State(S')
These relations are interpreted as follows: if we are in state S and event E occurs, we are to perform actions A and make a transition to state S'. Notice that S' can be equal to S.
As A and S' depend only on S and E, the kind of state machine described here is a Mealy Machine (see, for example, the corresponding Wikipedia article).
Like most gen_ behaviors, gen_statem keeps a server Data besides the state. Because of this, and as there is no restriction on the number of states (assuming that there is enough virtual machine memory) or on the number of distinct input events, a state machine implemented with this behavior is in fact Turing complete. But it feels mostly like an Event-Driven Mealy Machine.
3.2 Callback Modes
The gen_statem behavior supports two callback modes:
-
In mode state_functions, the state transition rules are written as some Erlang functions, which conform to the following convention:
StateName(EventType, EventContent, Data) -> ... code for actions here ... {next_state, NewStateName, NewData}.
This form is used in most examples here for example in section Example.
-
In mode handle_event_function, only one Erlang function provides all state transition rules:
handle_event(EventType, EventContent, State, Data) -> ... code for actions here ... {next_state, NewState, NewData}
See section One Event Handler for an example.
Both these modes allow other return tuples; see Module:StateName/3 in the gen_statem manual page. These other return tuples can, for example, stop the machine, execute state transition actions on the machine engine itself, and send replies.
Choosing the Callback Mode
The two callback modes give different possibilities and restrictions, but one goal remains: you want to handle all possible combinations of events and states.
This can be done, for example, by focusing on one state at the time and for every state ensure that all events are handled. Alternatively, you can focus on one event at the time and ensure that it is handled in every state. You can also use a mix of these strategies.
With state_functions, you are restricted to use atom-only states, and the gen_statem engine branches depending on state name for you. This encourages the callback module to gather the implementation of all event actions particular to one state in the same place in the code, hence to focus on one state at the time.
This mode fits well when you have a regular state diagram, like the ones in this chapter, which describes all events and actions belonging to a state visually around that state, and each state has its unique name.
With handle_event_function, you are free to mix strategies, as all events and states are handled in the same callback function.
This mode works equally well when you want to focus on one event at the time or on one state at the time, but function Module:handle_event/4 quickly grows too large to handle without branching to helper functions.
The mode enables the use of non-atom states, for example, complex states or even hierarchical states. If, for example, a state diagram is largely alike for the client side and the server side of a protocol, you can have a state {StateName,server} or {StateName,client}, and make StateName determine where in the code to handle most events in the state. The second element of the tuple is then used to select whether to handle special client-side or server-side events.
3.3 State Enter Calls
The gen_statem behavior can regardless of callback mode automatically call the state callback with special arguments whenever the state changes so you can write state entry actions near the rest of the state transition rules. It typically looks like this:
StateName(enter, _OldState, Data) -> ... code for state entry actions here ... {keep_state, NewData}; StateName(EventType, EventContent, Data) -> ... code for actions here ... {next_state, NewStateName, NewData}.
Depending on how your state machine is specified, this can be a very useful feature, but it forces you to handle the state enter calls in all states. See also the State Entry Actions chapter.
3.4 Actions
In the first section Event-Driven State Machines actions were mentioned as a part of the general state machine model. These general actions are implemented with the code that callback module gen_statem executes in an event-handling callback function before returning to the gen_statem engine.
There are more specific state-transition actions that a callback function can order the gen_statem engine to do after the callback function return. These are ordered by returning a list of actions in the return tuple from the callback function. These state transition actions affect the gen_statem engine itself and can do the following:
- Postpone the current event, see section Postponing Events
- Hibernate the gen_statem, treated in Hibernation
- Start a state time-out, read more in section State Time-Outs
- Start a generic time-out, read more in section Generic Time-Outs
- Start an event time-out, see more in section Event Time-Outs
- Reply to a caller, mentioned at the end of section All State Events
- Generate the next event to handle, see section Self-Generated Events
For details, see the gen_statem(3) manual page. You can, for example, reply to many callers, generate multiple next events, and set time-outs to relative or absolute times.
3.5 Event Types
Events are categorized in different event types. Events of all types are handled in the same callback function, for a given state, and the function gets EventType and EventContent as arguments.
The following is a complete list of event types and where they come from:
- cast
- Generated by gen_statem:cast.
- {call,From}
- Generated by gen_statem:call, where From is the reply address to use when replying either through the state transition action {reply,From,Msg} or by calling gen_statem:reply.
- info
- Generated by any regular process message sent to the gen_statem process.
- state_timeout
- Generated by state transition action {state_timeout,Time,EventContent} state timer timing out.
- {timeout,Name}
- Generated by state transition action {{timeout,Name},Time,EventContent} generic timer timing out.
- timeout
- Generated by state transition action {timeout,Time,EventContent} (or its short form Time) event timer timing out.
- internal
- Generated by state transition action {next_event,internal,EventContent}. All event types above can also be generated using {next_event,EventType,EventContent}.
3.6 Example
A door with a code lock can be seen as a state machine. Initially, the door is locked. When someone presses a button, an event is generated. Depending on what buttons have been pressed before, the sequence so far can be correct, incomplete, or wrong. If correct, the door is unlocked for 10 seconds (10,000 milliseconds). If incomplete, we wait for another button to be pressed. If wrong, we start all over, waiting for a new button sequence.
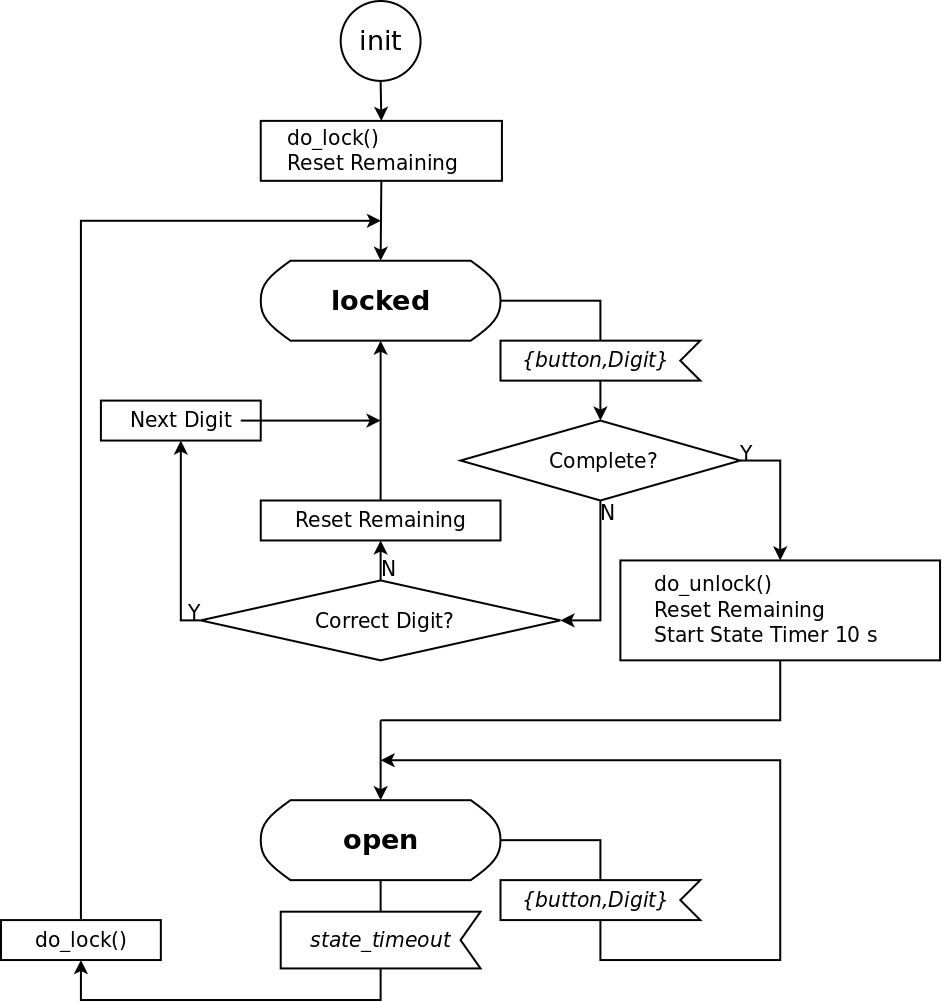
Figure 3.1: Code Lock State Diagram
This code lock state machine can be implemented using gen_statem with the following callback module:
-module(code_lock). -behaviour(gen_statem). -define(NAME, code_lock). -export([start_link/1]). -export([button/1]). -export([init/1,callback_mode/0,terminate/3,code_change/4]). -export([locked/3,open/3]). start_link(Code) -> gen_statem:start_link({local,?NAME}, ?MODULE, Code, []). button(Digit) -> gen_statem:cast(?NAME, {button,Digit}). init(Code) -> do_lock(), Data = #{code => Code, remaining => Code}, {ok, locked, Data}. callback_mode() -> state_functions. locked( cast, {button,Digit}, #{code := Code, remaining := Remaining} = Data) -> case Remaining of [Digit] -> do_unlock(), {next_state, open, Data#{remaining := Code}, [{state_timeout,10000,lock}]}; [Digit|Rest] -> % Incomplete {next_state, locked, Data#{remaining := Rest}}; _Wrong -> {next_state, locked, Data#{remaining := Code}} end. open(state_timeout, lock, Data) -> do_lock(), {next_state, locked, Data}; open(cast, {button,_}, Data) -> {next_state, open, Data}. do_lock() -> io:format("Lock~n", []). do_unlock() -> io:format("Unlock~n", []). terminate(_Reason, State, _Data) -> State =/= locked andalso do_lock(), ok. code_change(_Vsn, State, Data, _Extra) -> {ok, State, Data}.
The code is explained in the next sections.
3.7 Starting gen_statem
In the example in the previous section, gen_statem is started by calling code_lock:start_link(Code):
start_link(Code) -> gen_statem:start_link({local,?NAME}, ?MODULE, Code, []).
start_link calls function gen_statem:start_link/4, which spawns and links to a new process, a gen_statem.
-
The first argument, {local,?NAME}, specifies the name. In this case, the gen_statem is locally registered as code_lock through the macro ?NAME.
If the name is omitted, the gen_statem is not registered. Instead its pid must be used. The name can also be specified as {global,Name}, then the gen_statem is registered using global:register_name/2 in Kernel.
-
The second argument, ?MODULE, is the name of the callback module, that is, the module where the callback functions are located, which is this module.
The interface functions (start_link/1 and button/1) are located in the same module as the callback functions (init/1, locked/3, and open/3). It is normally good programming practice to have the client-side code and the server-side code contained in one module.
-
The third argument, Code, is a list of digits, which is the correct unlock code that is passed to callback function init/1.
-
The fourth argument, [], is a list of options. For the available options, see gen_statem:start_link/3.
If name registration succeeds, the new gen_statem process calls callback function code_lock:init(Code). This function is expected to return {ok, State, Data}, where State is the initial state of the gen_statem, in this case locked; assuming that the door is locked to begin with. Data is the internal server data of the gen_statem. Here the server data is a map with key code that stores the correct button sequence, and key remaining that stores the remaining correct button sequence (the same as the code to begin with).
init(Code) -> do_lock(), Data = #{code => Code, remaining => Code}, {ok,locked,Data}.
Function gen_statem:start_link is synchronous. It does not return until the gen_statem is initialized and is ready to receive events.
Function gen_statem:start_link must be used if the gen_statem is part of a supervision tree, that is, started by a supervisor. Another function, gen_statem:start can be used to start a standalone gen_statem, that is, a gen_statem that is not part of a supervision tree.
callback_mode() -> state_functions.
Function Module:callback_mode/0 selects the CallbackMode for the callback module, in this case state_functions. That is, each state has got its own handler function.
3.8 Handling Events
The function notifying the code lock about a button event is implemented using gen_statem:cast/2:
button(Digit) -> gen_statem:cast(?NAME, {button,Digit}).
The first argument is the name of the gen_statem and must agree with the name used to start it. So, we use the same macro ?NAME as when starting. {button,Digit} is the event content.
The event is made into a message and sent to the gen_statem. When the event is received, the gen_statem calls StateName(cast, Event, Data), which is expected to return a tuple {next_state, NewStateName, NewData}, or {next_state, NewStateName, NewData, Actions}. StateName is the name of the current state and NewStateName is the name of the next state to go to. NewData is a new value for the server data of the gen_statem, and Actions is a list of actions on the gen_statem engine.
locked( cast, {button,Digit}, #{code := Code, remaining := Remaining} = Data) -> case Remaining of [Digit] -> % Complete do_unlock(), {next_state, open, Data#{remaining := Code}, [{state_timeout,10000,lock}]}; [Digit|Rest] -> % Incomplete {next_state, locked, Data#{remaining := Rest}}; [_|_] -> % Wrong {next_state, locked, Data#{remaining := Code}} end. open(state_timeout, lock, Data) -> do_lock(), {next_state, locked, Data}; open(cast, {button,_}, Data) -> {next_state, open, Data}.
If the door is locked and a button is pressed, the pressed button is compared with the next correct button. Depending on the result, the door is either unlocked and the gen_statem goes to state open, or the door remains in state locked.
If the pressed button is incorrect, the server data restarts from the start of the code sequence.
If the whole code is correct, the server changes states to open.
In state open, a button event is ignored by staying in the same state. This can also be done by returning {keep_state, Data} or in this case since Data unchanged even by returning keep_state_and_data.
3.9 State Time-Outs
When a correct code has been given, the door is unlocked and the following tuple is returned from locked/2:
{next_state, open, Data#{remaining := Code}, [{state_timeout,10000,lock}]};
10,000 is a time-out value in milliseconds. After this time (10 seconds), a time-out occurs. Then, StateName(state_timeout, lock, Data) is called. The time-out occurs when the door has been in state open for 10 seconds. After that the door is locked again:
open(state_timeout, lock, Data) -> do_lock(), {next_state, locked, Data};
The timer for a state time-out is automatically cancelled when the state machine changes states. You can restart a state time-out by setting it to a new time, which cancels the running timer and starts a new. This implies that you can cancel a state time-out by restarting it with time infinity.
3.10 All State Events
Sometimes events can arrive in any state of the gen_statem. It is convenient to handle these in a common state handler function that all state functions call for events not specific to the state.
Consider a code_length/0 function that returns the length of the correct code (that should not be sensitive to reveal). We dispatch all events that are not state-specific to the common function handle_event/3:
... -export([button/1,code_length/0]). ... code_length() -> gen_statem:call(?NAME, code_length). ... locked(...) -> ... ; locked(EventType, EventContent, Data) -> handle_event(EventType, EventContent, Data). ... open(...) -> ... ; open(EventType, EventContent, Data) -> handle_event(EventType, EventContent, Data). handle_event({call,From}, code_length, #{code := Code} = Data) -> {keep_state, Data, [{reply,From,length(Code)}]}.
This example uses gen_statem:call/2, which waits for a reply from the server. The reply is sent with a {reply,From,Reply} tuple in an action list in the {keep_state, ...} tuple that retains the current state. This return form is convenient when you want to stay in the current state but do not know or care about what it is.
3.11 One Event Handler
If mode handle_event_function is used, all events are handled in Module:handle_event/4 and we can (but do not have to) use an event-centered approach where we first branch depending on event and then depending on state:
... -export([handle_event/4]). ... callback_mode() -> handle_event_function. handle_event(cast, {button,Digit}, State, #{code := Code} = Data) -> case State of locked -> case maps:get(remaining, Data) of [Digit] -> % Complete do_unlock(), {next_state, open, Data#{remaining := Code}, [{state_timeout,10000,lock}]}; [Digit|Rest] -> % Incomplete {keep_state, Data#{remaining := Rest}}; [_|_] -> % Wrong {keep_state, Data#{remaining := Code}} end; open -> keep_state_and_data end; handle_event(state_timeout, lock, open, Data) -> do_lock(), {next_state, locked, Data}. ...
3.12 Stopping
In a Supervision Tree
If the gen_statem is part of a supervision tree, no stop function is needed. The gen_statem is automatically terminated by its supervisor. Exactly how this is done is defined by a shutdown strategy set in the supervisor.
If it is necessary to clean up before termination, the shutdown strategy must be a time-out value and the gen_statem must in function init/1 set itself to trap exit signals by calling process_flag(trap_exit, true):
init(Args) -> process_flag(trap_exit, true), do_lock(), ...
When ordered to shut down, the gen_statem then calls callback function terminate(shutdown, State, Data).
In this example, function terminate/3 locks the door if it is open, so we do not accidentally leave the door open when the supervision tree terminates:
terminate(_Reason, State, _Data) -> State =/= locked andalso do_lock(), ok.
Standalone gen_statem
If the gen_statem is not part of a supervision tree, it can be stopped using gen_statem:stop, preferably through an API function:
... -export([start_link/1,stop/0]). ... stop() -> gen_statem:stop(?NAME).
This makes the gen_statem call callback function terminate/3 just like for a supervised server and waits for the process to terminate.
3.13 Event Time-Outs
A time-out feature inherited from gen_statem's predecessor gen_fsm, is an event time-out, that is, if an event arrives the timer is cancelled. You get either an event or a time-out, but not both.
It is ordered by the state transition action {timeout,Time,EventContent}, or just Time, or even just Time instead of an action list (the latter is a form inherited from gen_fsm.
This type of time-out is useful to for example act on inactivity. Let us restart the code sequence if no button is pressed for say 30 seconds:
... locked( timeout, _, #{code := Code, remaining := Remaining} = Data) -> {next_state, locked, Data#{remaining := Code}}; locked( cast, {button,Digit}, #{code := Code, remaining := Remaining} = Data) -> ... [Digit|Rest] -> % Incomplete {next_state, locked, Data#{remaining := Rest}, 30000}; ...
Whenever we receive a button event we start an event time-out of 30 seconds, and if we get an event type timeout we reset the remaining code sequence.
An event time-out is cancelled by any other event so you either get some other event or the time-out event. It is therefore not possible nor needed to cancel or restart an event time-out. Whatever event you act on has already cancelled the event time-out...
3.14 Generic Time-Outs
The previous example of state time-outs only work if the state machine stays in the same state during the time-out time. And event time-outs only work if no disturbing unrelated events occur.
You may want to start a timer in one state and respond to the time-out in another, maybe cancel the time-out without changing states, or perhaps run multiple time-outs in parallel. All this can be accomplished with generic time-outs. They may look a little bit like event time-outs but contain a name to allow for any number of them simultaneously and they are not automatically cancelled.
Here is how to accomplish the state time-out in the previous example by instead using a generic time-out named open_tm:
... locked( cast, {button,Digit}, #{code := Code, remaining := Remaining} = Data) -> case Remaining of [Digit] -> do_unlock(), {next_state, open, Data#{remaining := Code}, [{{timeout,open_tm},10000,lock}]}; ... open({timeout,open_tm}, lock, Data) -> do_lock(), {next_state,locked,Data}; open(cast, {button,_}, Data) -> {keep_state,Data}; ...
Just as state time-outs you can restart or cancel a specific generic time-out by setting it to a new time or infinity.
Another way to handle a late time-out can be to not cancel it, but to ignore it if it arrives in a state where it is known to be late.
3.15 Erlang Timers
The most versatile way to handle time-outs is to use Erlang Timers; see erlang:start_timer3,4. Most time-out tasks can be performed with the time-out features in gen_statem, but an example of one that can not is if you should need the return value from erlang:cancel_timer(Tref), that is; the remaining time of the timer.
Here is how to accomplish the state time-out in the previous example by instead using an Erlang Timer:
... locked( cast, {button,Digit}, #{code := Code, remaining := Remaining} = Data) -> case Remaining of [Digit] -> do_unlock(), Tref = erlang:start_timer(10000, self(), lock), {next_state, open, Data#{remaining := Code, timer => Tref}}; ... open(info, {timeout,Tref,lock}, #{timer := Tref} = Data) -> do_lock(), {next_state,locked,maps:remove(timer, Data)}; open(cast, {button,_}, Data) -> {keep_state,Data}; ...
Removing the timer key from the map when we change to state locked is not strictly necessary since we can only get into state open with an updated timer map value. But it can be nice to not have outdated values in the state Data!
If you need to cancel a timer because of some other event, you can use erlang:cancel_timer(Tref). Note that a time-out message cannot arrive after this, unless you have postponed it before (see the next section), so ensure that you do not accidentally postpone such messages. Also note that a time-out message may have arrived just before you cancelling it, so you may have to read out such a message from the process mailbox depending on the return value from erlang:cancel_timer(Tref).
Another way to handle a late time-out can be to not cancel it, but to ignore it if it arrives in a state where it is known to be late.
3.16 Postponing Events
If you want to ignore a particular event in the current state and handle it in a future state, you can postpone the event. A postponed event is retried after the state has changed, that is, OldState =/= NewState.
Postponing is ordered by the state transition action postpone.
In this example, instead of ignoring button events while in the open state, we can postpone them and they are queued and later handled in the locked state:
... open(cast, {button,_}, Data) -> {keep_state,Data,[postpone]}; ...
Since a postponed event is only retried after a state change, you have to think about where to keep a state data item. You can keep it in the server Data or in the State itself, for example by having two more or less identical states to keep a boolean value, or by using a complex state with callback mode handle_event_function. If a change in the value changes the set of events that is handled, then the value should be kept in the State. Otherwise no postponed events will be retried since only the server Data changes.
This is not important if you do not postpone events. But if you later decide to start postponing some events, then the design flaw of not having separate states when they should be, might become a hard to find bug.
Fuzzy State Diagrams
It is not uncommon that a state diagram does not specify how to handle events that are not illustrated in a particular state in the diagram. Hopefully this is described in an associated text or from the context.
Possible actions: ignore as in drop the event (maybe log it) or deal with the event in some other state as in postpone it.
Selective Receive
Erlang's selective receive statement is often used to describe simple state machine examples in straightforward Erlang code. The following is a possible implementation of the first example:
-module(code_lock). -define(NAME, code_lock_1). -export([start_link/1,button/1]). start_link(Code) -> spawn( fun () -> true = register(?NAME, self()), do_lock(), locked(Code, Code) end). button(Digit) -> ?NAME ! {button,Digit}. locked(Code, [Digit|Remaining]) -> receive {button,Digit} when Remaining =:= [] -> do_unlock(), open(Code); {button,Digit} -> locked(Code, Remaining); {button,_} -> locked(Code, Code) end. open(Code) -> receive after 10000 -> do_lock(), locked(Code, Code) end. do_lock() -> io:format("Locked~n", []). do_unlock() -> io:format("Open~n", []).
The selective receive in this case causes implicitly open to postpone any events to the locked state.
A selective receive cannot be used from a gen_statem behavior as for any gen_* behavior, as the receive statement is within the gen_* engine itself. It must be there because all sys compatible behaviors must respond to system messages and therefore do that in their engine receive loop, passing non-system messages to the callback module.
The state transition action postpone is designed to model selective receives. A selective receive implicitly postpones any not received events, but the postpone state transition action explicitly postpones one received event.
Both mechanisms have the same theoretical time and memory complexity, while the selective receive language construct has smaller constant factors.
3.17 State Entry Actions
Say you have a state machine specification that uses state entry actions. Allthough you can code this using self-generated events (described in the next section), especially if just one or a few states has got state entry actions, this is a perfect use case for the built in state enter calls.
You return a list containing state_enter from your callback_mode/0 function and the gen_statem engine will call your state callback once with the arguments (enter, OldState, ...) whenever the state changes. Then you just need to handle these event-like calls in all states.
... init(Code) -> process_flag(trap_exit, true), Data = #{code => Code}, {ok, locked, Data}. callback_mode() -> [state_functions,state_enter]. locked(enter, _OldState, Data) -> do_lock(), {keep_state,Data#{remaining => Code}}; locked( cast, {button,Digit}, #{code := Code, remaining := Remaining} = Data) -> case Remaining of [Digit] -> {next_state, open, Data}; ... open(enter, _OldState, _Data) -> do_unlock(), {keep_state_and_data, [{state_timeout,10000,lock}]}; open(state_timeout, lock, Data) -> {next_state, locked, Data}; ...
You can repeat the state entry code by returning one of {repeat_state, ...}, {repeat_state_and_data,_} or repeat_state_and_data that otherwise behaves exactly like their keep_state siblings. See the type state_callback_result() in the reference manual.
3.18 Self-Generated Events
It can sometimes be beneficial to be able to generate events to your own state machine. This can be done with the state transition action {next_event,EventType,EventContent}.
You can generate events of any existing type, but the internal type can only be generated through action next_event. Hence, it cannot come from an external source, so you can be certain that an internal event is an event from your state machine to itself.
One example for this is to pre-process incoming data, for example decrypting chunks or collecting characters up to a line break. Purists may argue that this should be modelled with a separate state machine that sends pre-processed events to the main state machine. But to decrease overhead the small pre-processing state machine can be implemented in the common state event handling of the main state machine using a few state data variables that then sends the pre-processed events as internal events to the main state machine.
The following example uses an input model where you give the lock characters with put_chars(Chars) and then call enter() to finish the input.
... -export(put_chars/1, enter/0). ... put_chars(Chars) when is_binary(Chars) -> gen_statem:call(?NAME, {chars,Chars}). enter() -> gen_statem:call(?NAME, enter). ... locked(enter, _OldState, Data) -> do_lock(), {keep_state,Data#{remaining => Code, buf => []}}; ... handle_event({call,From}, {chars,Chars}, #{buf := Buf} = Data) -> {keep_state, Data#{buf := [Chars|Buf], [{reply,From,ok}]}; handle_event({call,From}, enter, #{buf := Buf} = Data) -> Chars = unicode:characters_to_binary(lists:reverse(Buf)), try binary_to_integer(Chars) of Digit -> {keep_state, Data#{buf := []}, [{reply,From,ok}, {next_event,internal,{button,Chars}}]} catch error:badarg -> {keep_state, Data#{buf := []}, [{reply,From,{error,not_an_integer}}]} end; ...
If you start this program with code_lock:start([17]) you can unlock with code_lock:put_chars(<<"001">>), code_lock:put_chars(<<"7">>), code_lock:enter().
3.19 Example Revisited
This section includes the example after most of the mentioned modifications and some more using state enter calls, which deserves a new state diagram:
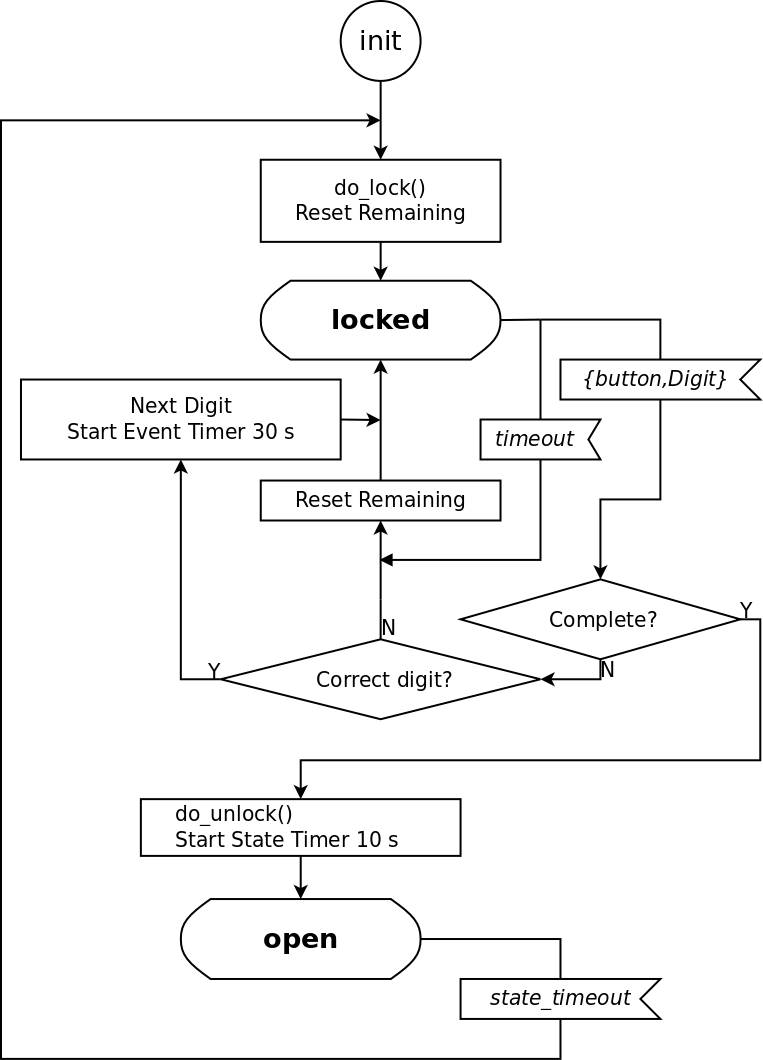
Figure 3.2: Code Lock State Diagram Revisited
Notice that this state diagram does not specify how to handle a button event in the state open. So, you need to read somewhere else that unspecified events must be ignored as in not consumed but handled in some other state. Also, the state diagram does not show that the code_length/0 call must be handled in every state.
Callback Mode: state_functions
Using state functions:
-module(code_lock). -behaviour(gen_statem). -define(NAME, code_lock_2). -export([start_link/1,stop/0]). -export([button/1,code_length/0]). -export([init/1,callback_mode/0,terminate/3,code_change/4]). -export([locked/3,open/3]). start_link(Code) -> gen_statem:start_link({local,?NAME}, ?MODULE, Code, []). stop() -> gen_statem:stop(?NAME). button(Digit) -> gen_statem:cast(?NAME, {button,Digit}). code_length() -> gen_statem:call(?NAME, code_length). init(Code) -> process_flag(trap_exit, true), Data = #{code => Code}, {ok, locked, Data}. callback_mode() -> [state_functions,state_enter]. locked(enter, _OldState, #{code := Code} = Data) -> do_lock(), {keep_state, Data#{remaining => Code}}; locked( timeout, _, #{code := Code, remaining := Remaining} = Data) -> {keep_state, Data#{remaining := Code}}; locked( cast, {button,Digit}, #{code := Code, remaining := Remaining} = Data) -> case Remaining of [Digit] -> % Complete {next_state, open, Data}; [Digit|Rest] -> % Incomplete {keep_state, Data#{remaining := Rest}, 30000}; [_|_] -> % Wrong {keep_state, Data#{remaining := Code}} end; locked(EventType, EventContent, Data) -> handle_event(EventType, EventContent, Data). open(enter, _OldState, _Data) -> do_unlock(), {keep_state_and_data, [{state_timeout,10000,lock}]}; open(state_timeout, lock, Data) -> {next_state, locked, Data}; open(cast, {button,_}, _) -> {keep_state_and_data, [postpone]}; open(EventType, EventContent, Data) -> handle_event(EventType, EventContent, Data). handle_event({call,From}, code_length, #{code := Code}) -> {keep_state_and_data, [{reply,From,length(Code)}]}. do_lock() -> io:format("Locked~n", []). do_unlock() -> io:format("Open~n", []). terminate(_Reason, State, _Data) -> State =/= locked andalso do_lock(), ok. code_change(_Vsn, State, Data, _Extra) -> {ok,State,Data}.
Callback Mode: handle_event_function
This section describes what to change in the example to use one handle_event/4 function. The previously used approach to first branch depending on event does not work that well here because of the state enter calls, so this example first branches depending on state:
... -export([handle_event/4]). ... callback_mode() -> [handle_event_function,state_enter]. %% State: locked handle_event( enter, _OldState, locked, #{code := Code} = Data) -> do_lock(), {keep_state, Data#{remaining => Code}}; handle_event( timeout, _, locked, #{code := Code, remaining := Remaining} = Data) -> {keep_state, Data#{remaining := Code}}; handle_event( cast, {button,Digit}, locked, #{code := Code, remaining := Remaining} = Data) -> case Remaining of [Digit] -> % Complete {next_state, open, Data}; [Digit|Rest] -> % Incomplete {keep_state, Data#{remaining := Rest}, 30000}; [_|_] -> % Wrong {keep_state, Data#{remaining := Code}} end; %% %% State: open handle_event(enter, _OldState, open, _Data) -> do_unlock(), {keep_state_and_data, [{state_timeout,10000,lock}]}; handle_event(state_timeout, lock, open, Data) -> {next_state, locked, Data}; handle_event(cast, {button,_}, open, _) -> {keep_state_and_data,[postpone]}; %% %% Any state handle_event({call,From}, code_length, _State, #{code := Code}) -> {keep_state_and_data, [{reply,From,length(Code)}]}. ...
Notice that postponing buttons from the locked state to the open state feels like a strange thing to do for a code lock, but it at least illustrates event postponing.
3.20 Filter the State
The example servers so far in this chapter print the full internal state in the error log, for example, when killed by an exit signal or because of an internal error. This state contains both the code lock code and which digits that remain to unlock.
This state data can be regarded as sensitive, and maybe not what you want in the error log because of some unpredictable event.
Another reason to filter the state can be that the state is too large to print, as it fills the error log with uninteresting details.
To avoid this, you can format the internal state that gets in the error log and gets returned from sys:get_status/1,2 by implementing function Module:format_status/2, for example like this:
... -export([init/1,terminate/3,code_change/4,format_status/2]). ... format_status(Opt, [_PDict,State,Data]) -> StateData = {State, maps:filter( fun (code, _) -> false; (remaining, _) -> false; (_, _) -> true end, Data)}, case Opt of terminate -> StateData; normal -> [{data,[{"State",StateData}]}] end.
It is not mandatory to implement a Module:format_status/2 function. If you do not, a default implementation is used that does the same as this example function without filtering the Data term, that is, StateData = {State,Data}, in this example containing sensitive information.
3.21 Complex State
The callback mode handle_event_function enables using a non-atom state as described in section Callback Modes, for example, a complex state term like a tuple.
One reason to use this is when you have a state item that when changed should cancel the state time-out, or one that affects the event handling in combination with postponing events. We will complicate the previous example by introducing a configurable lock button (this is the state item in question), which in the open state immediately locks the door, and an API function set_lock_button/1 to set the lock button.
Suppose now that we call set_lock_button while the door is open, and have already postponed a button event that until now was not the lock button. The sensible thing can be to say that the button was pressed too early so it is not to be recognized as the lock button. However, then it can be surprising that a button event that now is the lock button event arrives (as retried postponed) immediately after the state transits to locked.
So we make the button/1 function synchronous by using gen_statem:call and still postpone its events in the open state. Then a call to button/1 during the open state does not return until the state transits to locked, as it is there the event is handled and the reply is sent.
If a process now calls set_lock_button/1 to change the lock button while another process hangs in button/1 with the new lock button, it can be expected that the hanging lock button call immediately takes effect and locks the lock. Therefore, we make the current lock button a part of the state, so that when we change the lock button, the state changes and all postponed events are retried.
We define the state as {StateName,LockButton}, where StateName is as before and LockButton is the current lock button:
-module(code_lock). -behaviour(gen_statem). -define(NAME, code_lock_3). -export([start_link/2,stop/0]). -export([button/1,code_length/0,set_lock_button/1]). -export([init/1,callback_mode/0,terminate/3,code_change/4,format_status/2]). -export([handle_event/4]). start_link(Code, LockButton) -> gen_statem:start_link( {local,?NAME}, ?MODULE, {Code,LockButton}, []). stop() -> gen_statem:stop(?NAME). button(Digit) -> gen_statem:call(?NAME, {button,Digit}). code_length() -> gen_statem:call(?NAME, code_length). set_lock_button(LockButton) -> gen_statem:call(?NAME, {set_lock_button,LockButton}). init({Code,LockButton}) -> process_flag(trap_exit, true), Data = #{code => Code, remaining => undefined}, {ok, {locked,LockButton}, Data}. callback_mode() -> [handle_event_function,state_enter]. handle_event( {call,From}, {set_lock_button,NewLockButton}, {StateName,OldLockButton}, Data) -> {next_state, {StateName,NewLockButton}, Data, [{reply,From,OldLockButton}]}; handle_event( {call,From}, code_length, {_StateName,_LockButton}, #{code := Code}) -> {keep_state_and_data, [{reply,From,length(Code)}]}; %% %% State: locked handle_event( EventType, EventContent, {locked,LockButton}, #{code := Code, remaining := Remaining} = Data) -> case {EventType, EventContent} of {enter, _OldState} -> do_lock(), {keep_state, Data#{remaining := Code}}; {timeout, _} -> {keep_state, Data#{remaining := Code}}; {{call,From}, {button,Digit}} -> case Remaining of [Digit] -> % Complete {next_state, {open,LockButton}, Data, [{reply,From,ok}]}; [Digit|Rest] -> % Incomplete {keep_state, Data#{remaining := Rest}, [{reply,From,ok}, 30000]}; [_|_] -> % Wrong {keep_state, Data#{remaining := Code}, [{reply,From,ok}]} end end; %% %% State: open handle_event( EventType, EventContent, {open,LockButton}, Data) -> case {EventType, EventContent} of {enter, _OldState} -> do_unlock(), {keep_state_and_data, [{state_timeout,10000,lock}]}; {state_timeout, lock} -> {next_state, {locked,LockButton}, Data}; {{call,From}, {button,Digit}} -> if Digit =:= LockButton -> {next_state, {locked,LockButton}, Data, [{reply,From,locked}]}; true -> {keep_state_and_data, [postpone]} end end. do_lock() -> io:format("Locked~n", []). do_unlock() -> io:format("Open~n", []). terminate(_Reason, State, _Data) -> State =/= locked andalso do_lock(), ok. code_change(_Vsn, State, Data, _Extra) -> {ok,State,Data}. format_status(Opt, [_PDict,State,Data]) -> StateData = {State, maps:filter( fun (code, _) -> false; (remaining, _) -> false; (_, _) -> true end, Data)}, case Opt of terminate -> StateData; normal -> [{data,[{"State",StateData}]}] end.
It can be an ill-fitting model for a physical code lock that the button/1 call can hang until the lock is locked. But for an API in general it is not that strange.
3.22 Hibernation
If you have many servers in one node and they have some state(s) in their lifetime in which the servers can be expected to idle for a while, and the amount of heap memory all these servers need is a problem, then the memory footprint of a server can be mimimized by hibernating it through proc_lib:hibernate/3.
It is rather costly to hibernate a process; see erlang:hibernate/3. It is not something you want to do after every event.
We can in this example hibernate in the {open,_} state, because what normally occurs in that state is that the state time-out after a while triggers a transition to {locked,_}:
... %% State: open handle_event( EventType, EventContent, {open,LockButton}, Data) -> case {EventType, EventContent} of {enter, _OldState} -> do_unlock(), {keep_state_and_data, [{state_timeout,10000,lock},hibernate]}; ...
The atom hibernate in the action list on the last line when entering the {open,_} state is the only change. If any event arrives in the {open,_}, state, we do not bother to rehibernate, so the server stays awake after any event.
To change that we would need to insert action hibernate in more places. For example, for the state-independent set_lock_button and code_length operations that then would have to be aware of using hibernate while in the {open,_} state, which would clutter the code.
Another not uncommon scenario is to use the event time-out to triger hibernation after a certain time of inactivity.
This server probably does not use heap memory worth hibernating for. To gain anything from hibernation, your server would have to produce some garbage during callback execution, for which this example server can serve as a bad example.